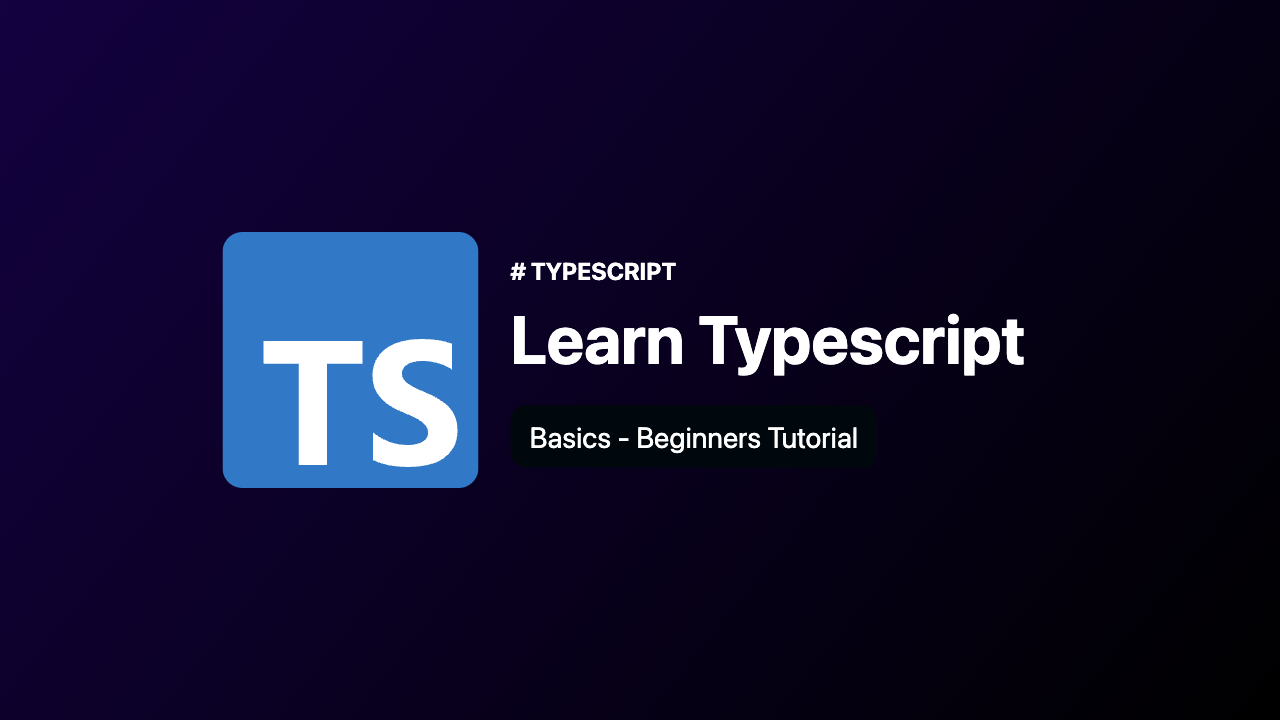
If you’re looking to enhance your web development skills, learning Typescript is a great place to start. This powerful superset of JavaScript offers a range of features and benefits that can help you write cleaner, more efficient code.
In this learn typescript basics beginners tutorial, we’ll cover the basics of installing Typescript and compiling it into JavaScript. We’ll also explore data types such as any types, built-in types, and user-defined types. Additionally, we’ll delve into object-oriented programming (OOP) concepts like classes and interfaces.
By the end of this tutorial, you’ll have a solid understanding of the fundamentals of Typescript and be well on your way to creating robust and scalable web applications. So let’s get started!
Installing Typescript
Before we dive into the specifics of Typescript, let’s first cover how to install it. The easiest way to get started with Typescript is to install it globally using npm. Open up your terminal and run the following command:
npm install -g typescript
This will install Typescript globally on your machine, allowing you to use it in any project.
Compiling Typescript
Once you have Typescript installed, you’ll need to compile it into JavaScript in order to run it in the browser. To do this, you can use the tsc
command, which stands for “Typescript compiler”.
First, create a new Typescript file with a .ts
extension. For example, you could create a file called app.ts
.
Next, open up your terminal and navigate to the directory where your app.ts
file is located. Then, run the following command:
tsc app.ts
This will compile your Typescript code into JavaScript and create a new file called app.js
.
Data Types in Typescript
One of the key benefits of Typescript is its support for static typing. This means that you can define the data types of your variables and functions, which can help catch errors before your code is even run.
Typescript supports a range of data types, including any types, built-in types, and user-defined types.
Any Types
The any
type is a special type in Typescript that can be used to represent any data type. This can be useful in situations where you don’t know the type of a variable ahead of time.
let myVariable: any = 'Hello, world!'
myVariable = 42
myVariable = true
Built-in Types
Typescript also supports a range of built-in data types, including:
number
: represents numeric valuesstring
: represents string valuesboolean
: represents boolean valuesnull
andundefined
: represent null and undefined values, respectively
let myNumber: number = 42
let myString: string = 'Hello, world!'
let myBoolean: boolean = true
let myNull: null = null
let myUndefined: undefined = undefined
User-defined Types
Finally, Typescript allows you to define your own custom data types using interfaces. Interfaces define a set of properties and methods that an object must have in order to be considered of that type.
interface Person {
name: string
age: number
sayHello(): void
}
class Employee implements Person {
name: string
age: number
constructor(name: string, age: number) {
this.name = name
this.age = age
}
sayHello() {
console.log(
`Hello, my name is ${this.name} and I am ${this.age} years old.`
)
}
}
const john = new Employee('John', 30)
john.sayHello() // Output: "Hello, my name is John and I am 30 years old."
Object-Oriented Programming in Typescript
Typescript also supports object-oriented programming (OOP) concepts like classes and interfaces.
Classes
Classes in Typescript are similar to classes in other object-oriented programming languages like Java or C#. They allow you to define a blueprint for creating objects with specific properties and methods.
class Person {
name: string
age: number
constructor(name: string, age: number) {
this.name = name
this.age = age
}
sayHello() {
console.log(
`Hello, my name is ${this.name} and I am ${this.age} years old.`
)
}
}
const john = new Person('John', 30)
john.sayHello() // Output: "Hello, my name is John and I am 30 years old."
Interfaces
As we saw earlier, interfaces allow you to define a set of properties and methods that an object must have in order to be considered of that type.
interface Person {
name: string
age: number
sayHello(): void
}
class Employee implements Person {
name: string
age: number
constructor(name: string, age: number) {
this.name = name
this.age = age
}
sayHello() {
console.log(
`Hello, my name is ${this.name} and I am ${this.age} years old.`
)
}
}
const john = new Employee('John', 30)
john.sayHello() // Output: "Hello, my name is John and I am 30 years old."
Conclusion
In this beginner’s tutorial, we covered the basics of Typescript, including how to install it, compile it into JavaScript, and use data types like any types, built-in types, and user-defined types. We also explored object-oriented programming concepts like classes and interfaces.
By now, you should have a solid understanding of the fundamentals of Typescript and be well on your way to creating robust and scalable web applications. Happy coding!