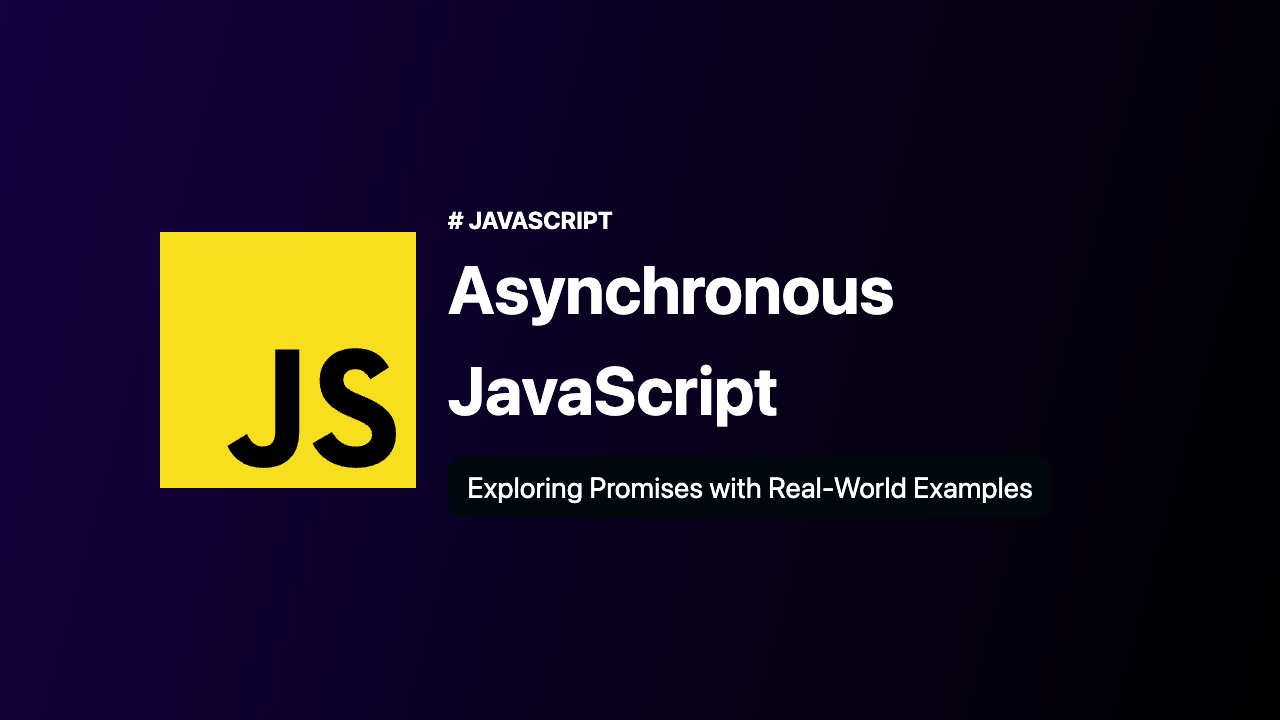
Introduction
Asynchronous programming is a crucial skill for any JavaScript developer. Promises, a feature introduced in ES6, have revolutionized how we handle asynchronous operations, making our code more readable and maintainable. In this blog post, we’ll dive into the world of Promises by exploring real-world examples that demonstrate their power and versatility.
Fetching Data from an API:
Consider a common scenario: fetching data from an API. Promises can streamline this process, making the code cleaner and more organized.
Example - Fetching Data with Promises:
const fetchWeatherData = (city) => {
const apiUrl = `https://api.weather.example.com/forecast/${city}`
return new Promise((resolve, reject) => {
fetch(apiUrl)
.then((response) => {
if (response.status === 200) {
resolve(response.json())
} else {
reject(new Error('Unable to fetch weather data.'))
}
})
.catch((error) => {
reject(error)
})
})
}
// Usage
fetchWeatherData('New York')
.then((data) => console.log(data))
.catch((error) => console.error(error))
Chaining Multiple Promises:
Promises can be easily chained to create a sequence of asynchronous operations. Let’s say we want to fetch weather data and then perform additional processing.
Example - Chaining Promises:
const fetchAndProcessWeather = (city) => {
fetchWeatherData(city)
.then((data) => {
// Perform additional processing on data
return processWeatherData(data)
})
.then((processedData) => {
console.log('Processed Weather Data:', processedData)
})
.catch((error) => {
console.error('An error occurred:', error)
})
}
// Usage
fetchAndProcessWeather('London')
Handling Parallel Promises:
In some cases, you might need to fetch data from multiple sources simultaneously. Promises can be used to handle parallel asynchronous tasks.
Example - Parallel Promises:
const fetchMultipleWeatherData = (cities) => {
const promises = cities.map((city) => fetchWeatherData(city))
Promise.all(promises)
.then((results) => {
console.log('Fetched Weather Data for Multiple Cities:', results)
})
.catch((error) => {
console.error('An error occurred:', error)
})
}
// Usage
fetchMultipleWeatherData(['New York', 'London', 'Tokyo'])
Conclusion
Promises are a powerful tool in modern JavaScript for handling asynchronous operations. Their clean and structured syntax simplifies complex workflows and enhances code readability. By understanding how to create, chain, and manage Promises, you’ll be better equipped to develop robust and responsive applications.
In this blog post, we explored real-world scenarios where Promises shine, from fetching data from APIs to handling parallel tasks. By incorporating Promises into your coding toolkit, you’ll take a significant step toward mastering asynchronous JavaScript and building more efficient and maintainable code.