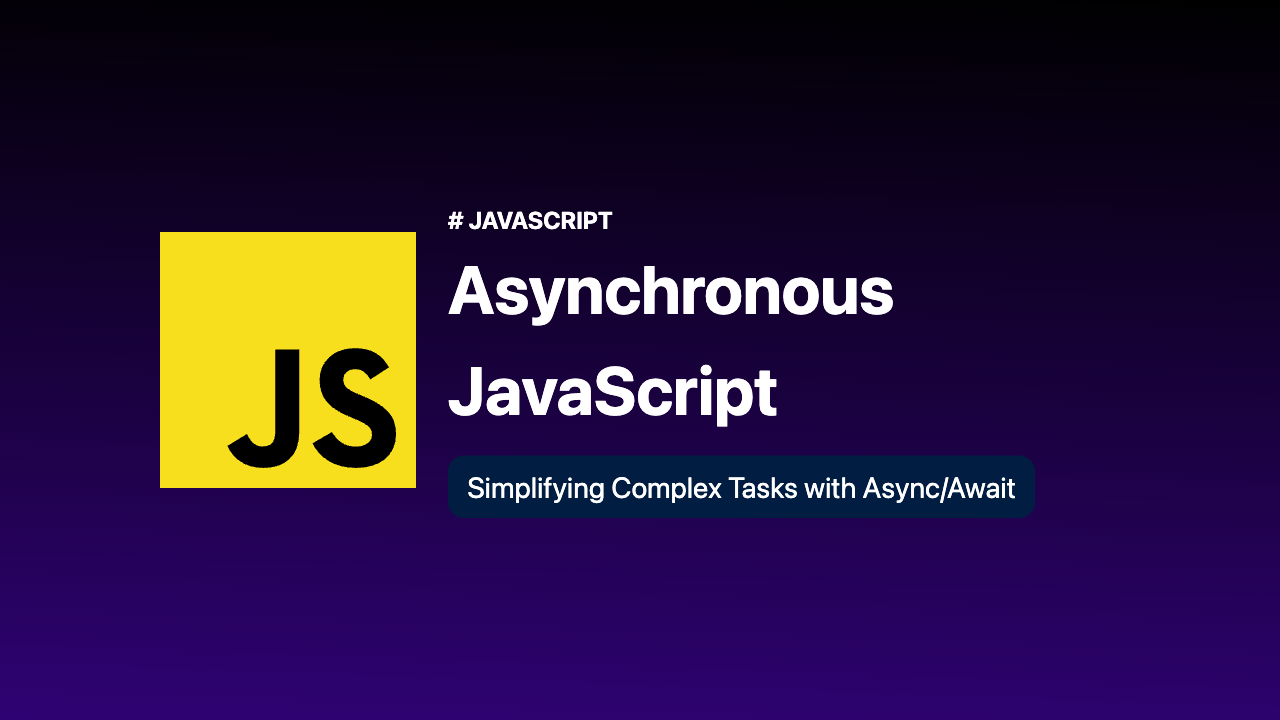
Introduction
Async/Await, introduced in ES2017, has become a game-changer in asynchronous programming. This feature allows developers to write asynchronous code in a more synchronous and readable manner. In this blog post, we’ll dive into the world of Async/Await by exploring practical examples that showcase its elegance and effectiveness.
Fetching Data from an API
Fetching data from an API is a common asynchronous operation. Async/Await makes this task more intuitive and concise.
Example - Fetching Data with Async/Await:
const fetchWeatherData = async (city) => {
const apiUrl = `https://api.weather.example.com/forecast/${city}`
try {
const response = await fetch(apiUrl)
if (response.status === 200) {
const data = await response.json()
return data
} else {
throw new Error('Unable to fetch weather data.')
}
} catch (error) {
throw error
}
}
// Usage
;(async () => {
try {
const data = await fetchWeatherData('New York')
console.log(data)
} catch (error) {
console.error(error)
}
})()
Chaining Multiple Async/Await Functions
Async/Await allows you to chain multiple asynchronous operations in a more linear fashion.
Example - Chaining Async Functions:
const fetchAndProcessWeather = async (city) => {
try {
const data = await fetchWeatherData(city)
const processedData = await processWeatherData(data)
console.log('Processed Weather Data:', processedData)
} catch (error) {
console.error('An error occurred:', error)
}
}
// Usage
fetchAndProcessWeather('London')
Handling Parallel Async Operations
Async/Await also simplifies handling multiple asynchronous tasks running in parallel.
Example - Handling Parallel Async Operations:
const fetchMultipleWeatherData = async (cities) => {
const promises = cities.map(async (city) => {
const data = await fetchWeatherData(city)
return data
})
try {
const results = await Promise.all(promises)
console.log('Fetched Weather Data for Multiple Cities:', results)
} catch (error) {
console.error('An error occurred:', error)
}
}
// Usage
fetchMultipleWeatherData(['New York', 'London', 'Tokyo'])
Conclusion:
Async/Await has transformed how developers approach asynchronous programming in JavaScript. Its clean and concise syntax makes complex tasks more manageable and readable. By incorporating Async/Await into your code, you’ll not only improve its clarity but also streamline error handling.
In this blog post, we explored practical examples that highlight Async/Await’s power, from fetching data from APIs to handling parallel tasks. Embrace this modern approach to asynchronous programming, and you’ll find yourself creating more maintainable, efficient, and responsive applications.