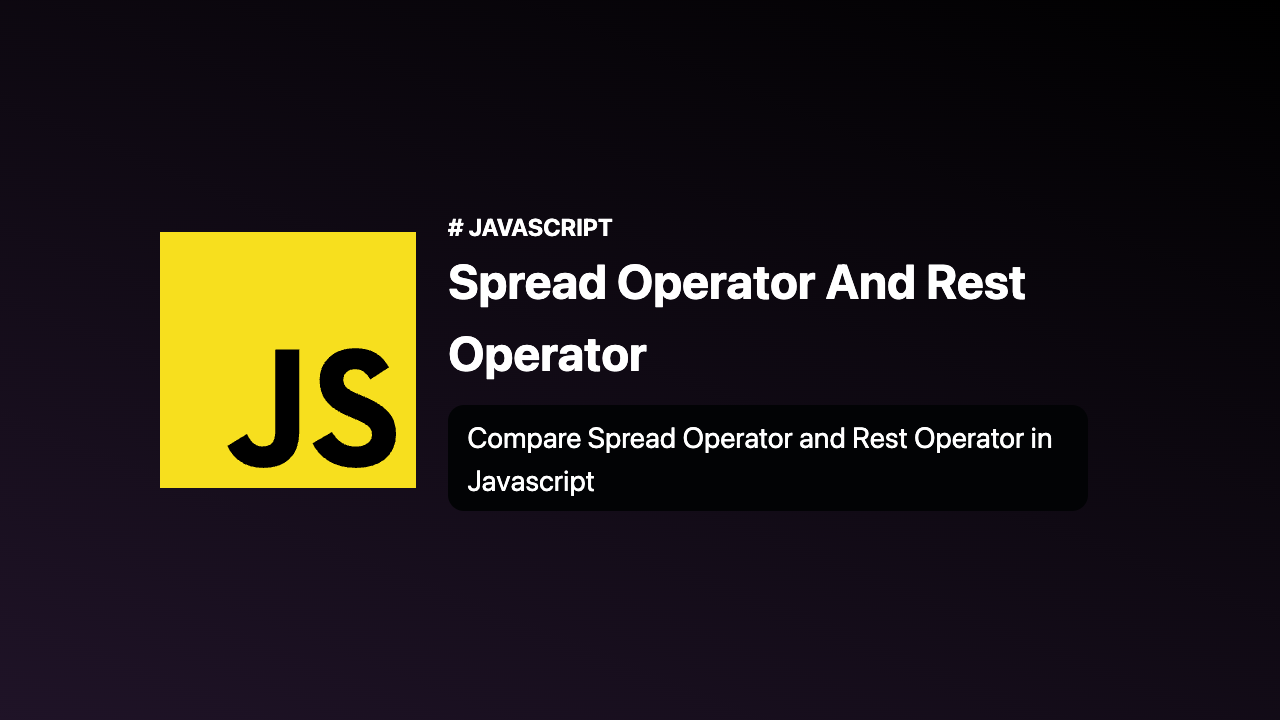
Introduction:
JavaScript, the backbone of modern web development, has evolved significantly over the years, introducing powerful features to enhance code readability and maintainability. Two such essential features are the Spread and Rest operators. In this blog, we will explore the Spread and Rest operators, understand their applications, and how they can elevate your JavaScript skills to the next level. Let’s dive in!
1. Understanding the Spread Operator:
The Spread operator, represented by three dots ( … ), allows us to expand elements from an array, object, or string into individual components. This operator simplifies the process of manipulating arrays and objects, making our code more concise and readable.
Use Cases:
1.1 Merging Arrays:
const array1 = [1, 2, 3]
const array2 = [4, 5, 6]
const mergedArray = [...array1, ...array2]
console.log(mergedArray) // Output: [1, 2, 3, 4, 5, 6]
1.2 Copying Arrays and Objects:
const originalArray = [1, 2, 3]
const copyOfArray = [...originalArray]
console.log(copyOfArray) // Output: [1, 2, 3]
const originalObject = { name: 'John', age: 30 }
const copyOfObject = { ...originalObject }
console.log(copyOfObject) // Output: { name: 'John', age: 30 }
1.3 Function Arguments:
const numbers = [1, 2, 3, 4, 5]
function sum(a, b, c, d, e) {
return a + b + c + d + e
}
const result = sum(...numbers)
console.log(result) // Output: 15
2. Exploring the Rest Operator:
The Rest operator, also represented by three dots ( … ), works in the opposite way to the Spread operator. It allows us to gather multiple elements into an array or object. This is especially useful when dealing with a variable number of function arguments.
Use Cases:
2.1 Gathering Function Arguments:
function displayNames(first, last, ...middle) {
console.log(`First Name: ${first}`)
console.log(`Last Name: ${last}`)
console.log(`Middle Names: ${middle.join(', ')}`)
}
displayNames('John', 'Doe', 'David', 'Michael', 'Smith')
/*
Output:
First Name: John
Last Name: Doe
Middle Names: David, Michael, Smith
*/
2.2 Collecting Remaining Array Elements:
const fruits = ['apple', 'banana', 'orange', 'grape']
const [firstFruit, ...remainingFruits] = fruits
console.log(firstFruit) // Output: apple
console.log(remainingFruits) // Output: ['banana', 'orange', 'grape']
Conclusion:
The Spread and Rest operators are powerful tools that simplify working with arrays and objects in JavaScript. The Spread operator allows us to merge arrays, copy objects, and handle function arguments effortlessly. On the other hand, the Rest operator enables us to gather function arguments dynamically and collect remaining array elements with ease.
By leveraging these operators, you can write more concise, readable, and maintainable code. So, embrace the power of the Spread and Rest operators and take your JavaScript skills to new heights! Happy coding!